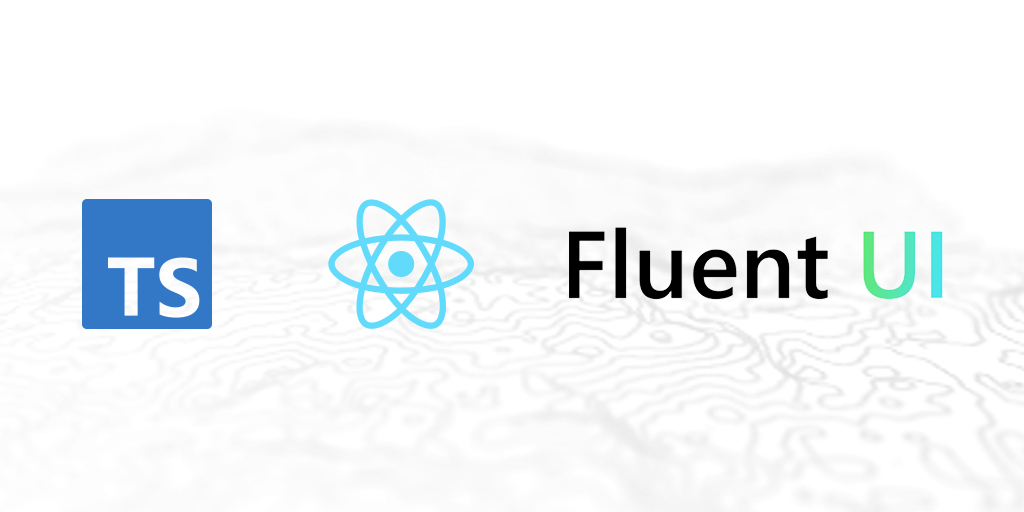
Choosing what platform to build your new app in is always a challenge. Usually, you’ll default to what you know, be that Angular, React, Vue. etc. Sometimes that decision is made for you, sometimes, if you’re lucky, you can define it for yourself. In my case using TypeScript, React and Fluent UI to build applications has been the best development decision I’ve made in a long time.
TypeScript
In recent years, under Microsoft’s stewardship, TypeScript has filled a void in the JavaScript dev world. Their mandate is simple; Add types to JavaScript. Being an untyped language makes JavaScript extremely flexible, but also extremely hard to debug and refactor. It’s easy for simple errors to sneak in.
For example in JavaScript:
let foo = 'bar';
... some other code
foo = true;
JavaScript will happily allow that, and you could get unexpected results.
Using TypeScript:
let foo:string = 'bar';
... some other code
foo = true;
You get a useful Type 'boolean' is not assignable to type 'string'.
error. Nice. It’s caught before you ever think of deploying.
React / Hooks
Ever since Facebook created React, it’s been a hit in the dev world. Virtual dom and state management take away the tedious task of updating the dom yourself. You never need to question if your DOM is up to date. Just make sure your state is correct, and your DOM will follow.
It wasn’t always as cut and dry. When React started they only had a single shared state
object for each component. That could get updated from anywhere in your component via setState
. With some discipline managing that is possible. However along the way various “lifecycle” methods were added to cover use cases that keep coming up in real world applications. So now was your state could be updated in a variety of methods, like didComponentReceiveProps()
or getDerivedStateFromProps()
. For larger apps the state could quickly became unmanageable and errors would creep in. If you learned and understood the component lifecycle things were generally OK. Even then, it was very easy to get lost in the maze of those lifecycle methods. This is where hooks come into the picture.
Old class style
export default class MyName extends React.Component {
constructor(props) {
super(props);
this.state = {name: ''};
}
function clickHandler() {
this.setState({name: 'My Name'});
}
render() {
return <p>{this.state.name}</p>;
}
}
Functional using Hooks
export cost MyName = () => {
const [name, setName] = useState('');
const onSomeButtonClick = useCallback(() => {
setName('My Name');
}, []);
return (
<p>{name}</p>
);
}
In this simple example, we have distilled our name
value into a single state value. We also get a single method to update that state. When that value changes it displays your name on the dom. Easy. All that logic to update the innerHTML, or create new dom elements, is gone. You only need to worry about your app state and the business logic to update that state. You can actually still use classes since Facebook has no plans to remove support for classes yet. For new applications, it’s recommended to use hooks. Hooks do have their own set of rules. However there is fantastic eslint support, that will check for those rules for you. In addition, React will surface useful errors to you if something does make it past your linter.
Fluent UI
Some of you are probably wondering to yourself “Why does a Microsoft framework fall into this list.” Hear me out.
- Microsoft builds and maintains TypeScript. Already a dev staple. Fluent UI is actually built-in TypeScript. So you immediately get all the benefits that TypeScript offers.
- Microsoft uses this framework for their own Office apps. So it’s constantly updated and bug fixed.
- It’s a complete component set. Need a List, CheckBox, RadioButton, (or as MS calls them a ChoiceGroup), Tooltip or a Dialog? They have that. Need theming? They also have that.
- Built for React.
- It’s very well-architected, everything has a clear purpose. For example to get more control over selection or focus you would use a
<Selection />
or<FocusZone />
control, instead of accessing those values from the controls. - Being well-architected it’s also very flexible. You can use just a Button if that’s all you need.
- Styling is also very flexible. You can use their built-in JSCSS & theming. Or use SASS (my preferred method). All the components have consistently named CSS classes attached. Ex;
.ms-Button
or.ms-Checkbox
That just means if you need to style outside what their theme allows, it’s totally possible.
For any framework choice, keep in mind your target. My main experience is with Fluent UI for the web, which is built for desktop-style applications (Think Office). They’re also working on providing controls for all other platforms. At the time of this writing, the web component set is the most complete. However there is active development on the other platforms, so the future looks bright for utilizing Fluent UI for your entire application stack.
Resources
TypeScript: https://www.typescriptlang.org/
React: https://reactjs.org/
Fluent UI for Web: https://developer.microsoft.com/en-us/fluentui#/controls/web
I’ll definitely be checking out Fluent UI – thank you for the blog post!
I’m curious how you are building project’s with this stack.
Webpack? We are wanting to compile *TO* ES6, but we haven’t arrived at a good solution yet. We avoid async/await in our front end because of this limitation and I’m really hoping we can discover a solid build solution.
Our project is TS, React, LESS, and likely soon to include Fluent UI.
I’m curious what your take is on styled components (styles in TS for React) vs SCSS / LESS.
It seems like a big scary React-only step to take – codifying all styling in TS.
Thanks for any insights,
Keldon Rush
@Keldon Rush
Thanks for reading!
For building; I’ve been using webpack to manage that process. There are some newer tools like Snowpack or esbuild that would also work, but are less porven. In one project it was a custom webpack setup. For a quicker setup, that doesn’t require as much customization, I prefer using create-react-app (which still uses webpack in the background). Both can be configured to compile to ES6 or ES5 based on your needs.
SASS/SCSS is my preference for styling, it has the most features. imho its the most robust styling system out there. Using webpack, it handles all the sass (or less) compiling for you, which is fantastic. With `import ./YourStyle.scss` there is a small coupling to your TS, but imho its light enough that it’s not a concern.
I’m curious how you are building project’s with this stack.
Thank you for the blog post!
I will definitely review the UI fluid: Thank you for the publication of the blog! I am curious how you are building the project with this pile.
I didn’t have any expectations concerning that title, but the more I was astonished. The author did a great job. I spent a few minutes reading and checking the facts. Everything is very clear and understandable. I like posts that fill in your knowledge gaps. This one is of the sort.
Thank you for this article. This really helped me.
Great post! Thanks for Sharing.