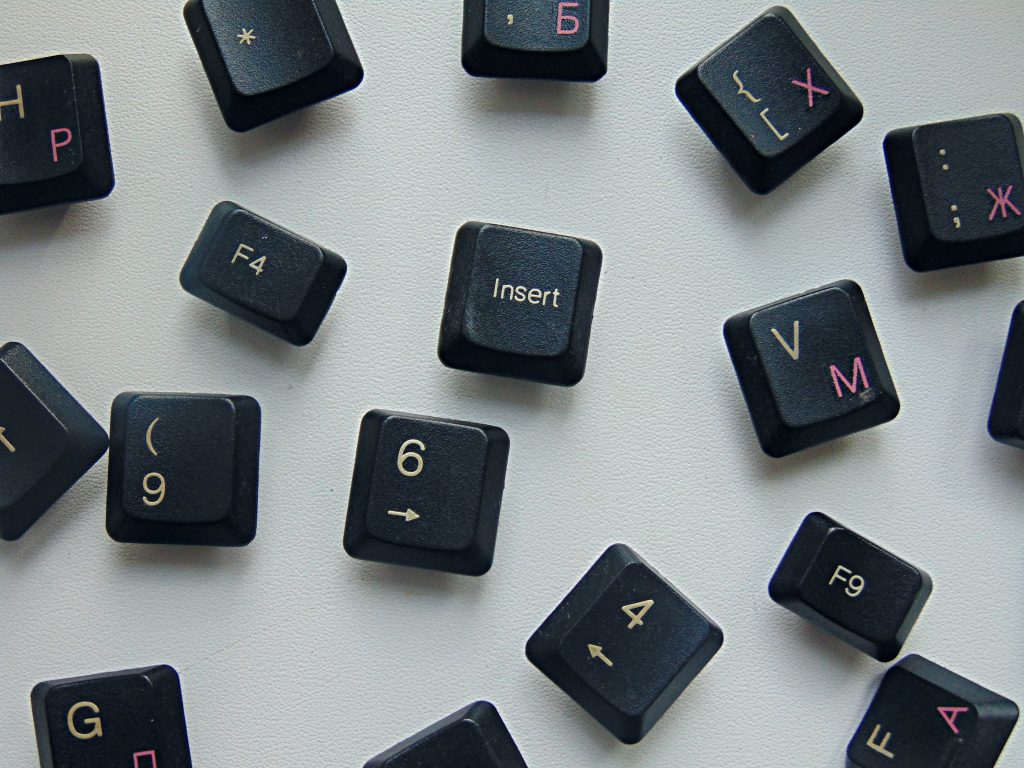
In a previous post we looked at a method for binding directly to the keyboard. While this is handy for quick spikes, or truly global listeners, it is a little dangerous as it is easy to forget to remove these listeners. In this post we’ll look at a couple of different ways to do this a little more safely.
For more complex use cases Flutter provides a Shortcuts/Actions/ Intents system, which is quite powerful, but can be hard to work with for simple use cases. Requiring the creation of several classes and significant boilerplate to accomplish simple tasks. Often you are left wishing there was something simpler.
That’s where the CallbackShortcuts widget comes in. Rather than creating Intents
and Actions
and binding them all together, you can just provide some keys combos and a method to trigger:
return CallbackShortcuts( bindings: { const SingleActivator(LogicalKeyboardKey.keyR): _handleResetPressed, }, child: Focus( autofocus: true, child: SomePageInYourApp(), ), );
Here we use the SingleActivator class to bind our _handleResetPressed
method to the “R” key SingleActivator(LogicalKeyboardKey.keyR).
Notice that a Focus
widget with autoFocus: true
is also required. If the current focus is not below the shortcuts widget, the callback will not be triggered.
If you’re wondering what SingleActivator
is, it’s just a convenience class Flutter gives you to express basic key bindings. It supports the common modifiers of: control, shift, alt and meta. So for example, you could bind to [R + Ctrl + Shift] very easily:
SingleActivator(LogicalKeyboardKey.keyR, shift: true, control: true)
There are a couple of other types of activators provided by Flutter:
- CharacterActivator – A shortcut combination that is mapped to a specific character rather than a specific set of keys. For example you may want to listen for the “?” character, and depending on the locale the key combinations to produce this character might differ.
- LogicalKeySet – An alternative way to express key mappings. Useful for advanced key combinations or sequences.
The nice thing about using the Shortcuts
or CallbackShortcuts
widget is that your listeners will be automatically cleaned up when the view is disposed. On the other hand, you now have the problem of Focus to deal with.
If this post was helpful, or you have any questions or feedback, please let us know below!
Thanks bro!
Awesome stuff!!!