One of the most verbose parts of Flutter is handling of various font styles, sizes, and families. In this post, we’ll show a couple of the tricks we’re using in production to ease this pain point.
Styles.dart
The first step we do in nearly every project, is to define a base Styles file, that will hold a variety of small classes, defining our core Fonts and TextStyles:
class Fonts {
static String get body => "Lato";
static String get title => "Roboto";
}
class FontSizes {
static double scale = 1;
static double get body => 14 * scale;
static double get bodySm => 12 * scale;
static double get title => 16 * scale;
}
class TextStyles {
static TextStyle get bodyFont => TextStyle(fontFamily: Fonts.body);
static TextStyle get titleFont => TextStyle(fontFamily: Fonts.title);
static TextStyle get title => titleFont.copyWith(fontSize: FontSizes.title);
static TextStyle get titleLight => title.copyWith(fontWeight: FontWeight.w300);
static TextStyle get body => bodyFont.copyWith(fontSize: FontSizes.body, fontWeight: FontWeight.w300);
static TextStyle get bodySm => body.copyWith(fontSize: FontSizes.bodySm);
}
Some things to note:
- Why the get functions instead of variables? Hot Reload! By using static functions, we can change these values at run-time for extremely fast iteration and tweaking.
- We can change the font style, or font sizes globally, extremely easily and quickly. All styling code is consolidated in one tight package.
- FontSizes.scale – A bit outside the scope of this article, but we like to expose a global scale modifier to our fonts. Normally this is 1, but we can tweak it for different form factors.
With the above class, we can now define a Text widget like so:
Text("I am Text.", style: TextStyles.body);
That’s not only a lot more readable, it’s also is significantly easier to maintain as your project scales.
Ok, so that’s pretty good… but it can be better!
While the above approach is an excellent foundation, it could still spiral out of control if you tried to define every single combination of bold/italic/character spacing. There can be a lot of ‘one off’ styles in an app, and it would be nice to be able to easily tweak these styles on the fly…
Dart Extensions to the rescue!
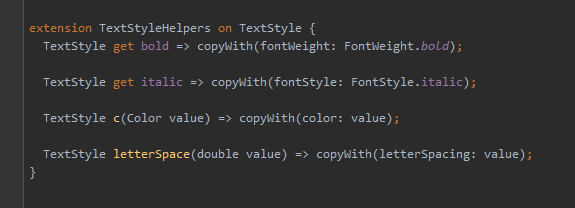
Modifying a text style is actually pretty easy, using the copyWith API, it’s just kinda verbose:
TextStyles.body.copyWith(fontWeight: FontWeight.bold, fontStyle: FontStyle.italic, letterSpacing: 1.6)
Oof. That’s a lot of code to just add bold, italic and tweak the letter spacing. It’s not super readable either, the important stuff (body, bold, italic, 1.6) represent only 15% of the characters, the rest is boilerplate.
We define a super simple TextStyle Extension to ease this pain:
extension TextStyleHelpers on TextStyle {
TextStyle get bold => copyWith(fontWeight: FontWeight.bold);
TextStyle get italic => copyWith(fontStyle: FontStyle.italic);
TextStyle letterSpace(double value) => copyWith(letterSpacing: value);
}
Now, we can re-write it like this:
TextStyles.body.bold.italic.letterSpace(1.6)
Much better! Boilerplate is only about 50% of the character count, and the intent is much more clear.
We thought of creating a package for this, but decided that it should probably live in the excellent styled_widget package, so we’ll try and make that happen soon.
Thanks for checking out the post!
Love this — it’d make more sense as it’s own package IMO. I’d love to use this without subscribing to the whole styled_widget mantra.
Good point. And I guess if we create it as a package, Division or StyledWidget can always just add it as a dependancy. We’ll consider it!
Shawn, this is exactly what I was needing to do. It seems, however, that this doesn’t work with the newest flutter/dart perhaps? I am getting an error that ‘copyWith’ is not defined for class ‘TextStyle’. Wondering if you or anyone else was seeing the same as me.
whats wrong using theme api ?
How would this support dark/light theme?
We keep our Colors in a separate Theme class, and apply that to the Material app, which takes care of changing most texfields to black/white depending on brightness.
In our theme class we also have like `mainTextColor` and `inverseTextColor` that our views can use, which are black and white, but depending on brightness one or the other will be ‘main’.
I see! Thanks for the reply 🙂
Here’s a post on that:
https://blog.gskinner.com/archives/2020/04/flutter-create-custom-app-themes.html
Basic idea is make your own theme class, whatever matches your design, then have a `toThemeData` method, that can convert your theme into the Material Theme, and pass that into MaterialApp.
Then your views can use your AppTheme, while MaterialApp will take care of auto-styling the material widgets.